The SQL Operators
With SQL queries, several operations are carried out on the data using SQL operators. Many SQL operators, such as SQL arithmetic operators, SQL comparison operators, SQL logical operators, SQL String Concatenation operator, SQL Compound Operators, SQL Bitwise Operators, and others, can be used to group them together.
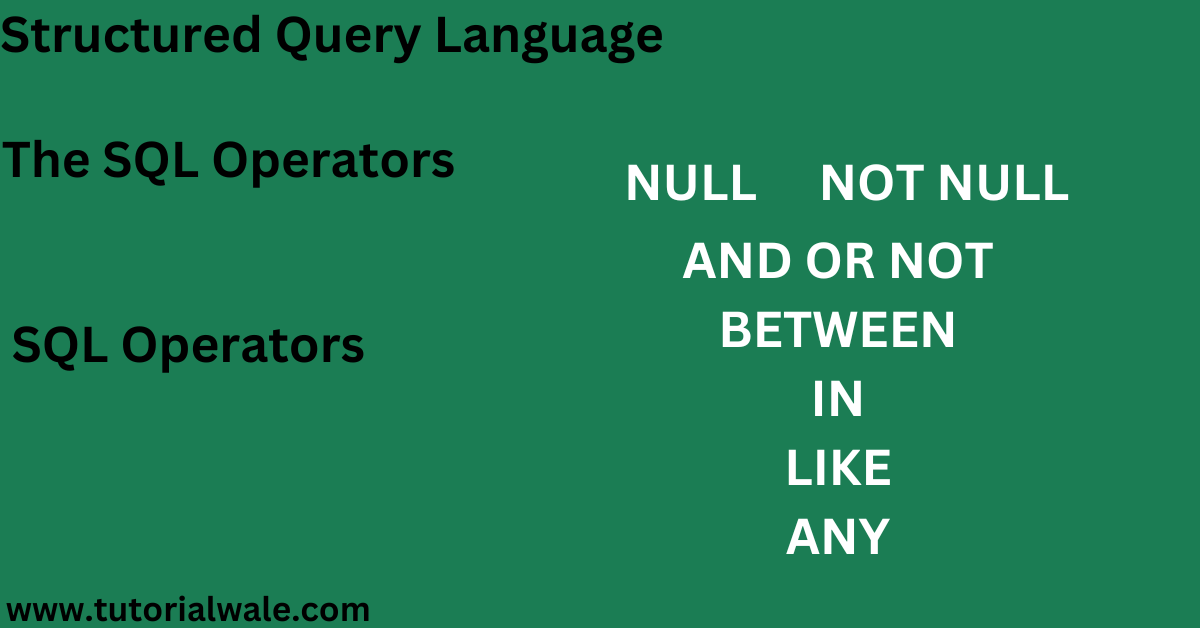
What are SQL operators?
The SQL operators are special symbols or keywords or character used to perform various operations on data within SQL queries.
These operations include SQL arithmetic calculations, SQL logical comparisons, and SQL string manipulations, and more. SQL operators allow you to filter, manipulate, and transform data in your database tables.
Here are some syntex of each type of SQL operator:
SQL Arithmetic Operators
Mathematical operations on numerical data are carried out using arithmetic operators.
- Addition (+)
- Subtraction (-)
- Multiplication (*)
- Division (/)
- Modulus (%)
Example of SQL Arithmetic Operators:
SELECT Salary + Bonus AS TotalIncome
FROM Employee;
In above example, the addition operator (+) is used to calculate the total income by adding the “Salary” and “Bonus” columns.
SQL Comparison Operators
Comparison operators are used to compare values in SQL queries and return Boolean results.
- Equal to (=)
- Not equal to (<> or !=)
- Greater than (>)
- Less than (<) Greater than or equal to (>=)
- Less than or equal to (<=)
Example of SQL Comparison Operators:
SELECT ProductName, Price
FROM Products
WHERE Price >= 50;
In above example, the greater than or equal to operator (>=) is used to filter products with a price greater than or equal to 50.
SQL Logical Operators
SQL Logical operators are used to combine or negate conditions in SQL queries.
- AND
- OR
- NOT
- BETWEEN
- IN
- LIKE
- ANY
Example of SQL Logical Operators:
SELECT ProductName, Price
FROM Products
WHERE Category = ‘Electronics’ AND Price < 100;
The AND operator is used to filter products that belong to the ‘Electronics’ category and have a price less than 100.
SQL String Concatenation Operator
The string concatenation operator (||) is used to combine two or more strings.
Example of SQL String Concatenation Operator:
SELECT FirstName || ‘ ‘ || LastName AS FullName
FROM Employees;
The || operator is used to concatenate the “FirstName” and “LastName” columns to create a full name.
SQL Assignment Operator
The assignment operator (=) is used to assign values to variables or columns.
Example of SQL Assignment Operator:
UPDATE Employees
SET Salary = Salary * 1.1
WHERE Department = ‘Sales’;
In this example, the = operator is used to assign a new salary to employees in the ‘Sales’ department.
SQL NULL Comparison Operators
SQL provides special operators for working with NULL values.
- IS NULL
- IS NOT NULL
Example of NULL Comparison Operators:
SELECT ProductName
FROM Products
WHERE Category IS NULL;
In this example, the IS NULL operator is used to find products with no assigned category.
SQL Compound Operators
- Add equals (+=)
- Subtract equals (-=)
- Modulus equals (%=)
- Multiple equals (*=)
- Divide equals (/=)
- AND equals (&=)
SQL Bitwise Operators
- & AND Bitwise
- | OR Bitwise
- ^ OR Bitwise
These are a few of the typical SQL operators used in SQL queries to alter data, compare values, and execute various actions on it. For filtering, calculating, and changing data within a database, operators are essential in SQL.